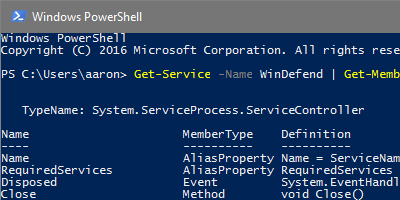
Learn how to discover all of a PowerShell object’s properties and see their values.
Object properties tell us about the object
Every time you run a “Get-” PowerShell cmdlet, you receive a single object or set of objects. In the programming world, an object’s properties are attributes about the object itself. A property could be a text string, a number, a timestamp, or any other descriptive value. Each property has a name and a corresponding value (and that value could potentially be null).
Not all properties displayed by default
Depending on the object type, not all of a object’s properties are displayed when you run the “Get-” cmdlet and display the output. If the output uses Format-Table, trying to squeeze all of the properties into the table could make the output noisy and unreadable, so it makes sense that some properties are excluded. However even if you use Format-List, some properties may still not be shown by default.
For demonstration, we’ll run Get-Service for the Windows Defender service and look at the default output.
PS C:\Users\aaron> Get-Service -Name WinDefend Status Name DisplayName ------ ---- ----------- Stopped WinDefend Windows Defender Service
Note that only three properties are displayed: Status, Name, and DisplayName. Are those the only properties of the object? Let’s format the output using Format-List.
PS C:\Users\aaron> Get-Service -Name WinDefend | Format-List Name : WinDefend DisplayName : Windows Defender Service Status : Stopped DependentServices : {} ServicesDependedOn : {RpcSs} CanPauseAndContinue : False CanShutdown : False CanStop : False ServiceType : Win32OwnProcess
Now nine properties are displayed; six more than the default output! That must be all of the properties then, right? How can we tell for sure?
Use Get-Member to see an object’s properties and methods
The Get-Member cmdlet is used to definitively show us a PowerShell object’s defined properties and methods. We use it by piping the output from our Get-Service cmdlet into Get-Member. Note in the sample output below the TypeName value at the top: System.ServiceProcess.ServiceController. This Type is actually a .NET class. If you do a web search for the TypeName, one of your first search results will be this MSDN documentation page. You can match up the Properties and Methods listed in the output below with the MSDN documentation, which includes additional descriptive text about each property and method.
PS C:\Users\aaron> Get-Service -Name WinDefend | Get-Member TypeName: System.ServiceProcess.ServiceController Name MemberType Definition ---- ---------- ---------- Name AliasProperty Name = ServiceName RequiredServices AliasProperty RequiredServices = ServicesDependedOn Disposed Event System.EventHandler Disposed(System.Object, System.EventArgs) Close Method void Close() Continue Method void Continue() CreateObjRef Method System.Runtime.Remoting.ObjRef CreateObjRef(type requestedType) Dispose Method void Dispose(), void IDisposable.Dispose() Equals Method bool Equals(System.Object obj) ExecuteCommand Method void ExecuteCommand(int command) GetHashCode Method int GetHashCode() GetLifetimeService Method System.Object GetLifetimeService() GetType Method type GetType() InitializeLifetimeService Method System.Object InitializeLifetimeService() Pause Method void Pause() Refresh Method void Refresh() Start Method void Start(), void Start(string[] args) Stop Method void Stop() WaitForStatus Method void WaitForStatus(System.ServiceProcess.ServiceControllerStatus desiredStatus), void WaitForStatus(System.ServiceProcess.ServiceControllerStatus desiredStatus, timespan tim... CanPauseAndContinue Property bool CanPauseAndContinue {get;} CanShutdown Property bool CanShutdown {get;} CanStop Property bool CanStop {get;} Container Property System.ComponentModel.IContainer Container {get;} DependentServices Property System.ServiceProcess.ServiceController[] DependentServices {get;} DisplayName Property string DisplayName {get;set;} MachineName Property string MachineName {get;set;} ServiceHandle Property System.Runtime.InteropServices.SafeHandle ServiceHandle {get;} ServiceName Property string ServiceName {get;set;} ServicesDependedOn Property System.ServiceProcess.ServiceController[] ServicesDependedOn {get;} ServiceType Property System.ServiceProcess.ServiceType ServiceType {get;} Site Property System.ComponentModel.ISite Site {get;set;} StartType Property System.ServiceProcess.ServiceStartMode StartType {get;} Status Property System.ServiceProcess.ServiceControllerStatus Status {get;} ToString ScriptMethod System.Object ToString();
Display all PowerShell object’s properties in output
From the Get-Member output, we learn there are fourteen properties and two alias properties of the System.ServiceProcess.ServiceController object type. However if you recall, when we used Format-List to display the output, we were only shown nine properties. To display ALL of the properties, we need to use the -Property parameter with a wildcard value. You see below sixteen properties are displayed, even if they have null values. This approach is helpful when you exploring results and are looking for properties of interest. Get-Member and MSDN documentation may give you an idea of what properties are helpful to you, but interrogating a sample object directly using -Property * will help you validate that a property has the information you need.
PS C:\Users\aaron> Get-Service -Name WinDefend | Format-List -Property * Name : WinDefend RequiredServices : {RpcSs} CanPauseAndContinue : False CanShutdown : False CanStop : False DisplayName : Windows Defender Service DependentServices : {} MachineName : . ServiceName : WinDefend ServicesDependedOn : {RpcSs} ServiceHandle : Status : Stopped ServiceType : Win32OwnProcess StartType : Manual Site : Container :