JSON (JavaScript Object Notation) has become a standard format for data exchange due to its lightweight nature and compatibility with many languages, including PowerShell. This article provides a comprehensive guide to handling JSON in PowerShell.
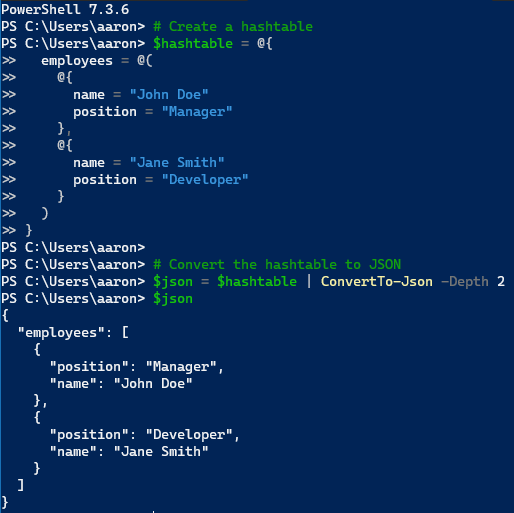
Understanding JSON
JSON is a data format that uses human-readable text to represent data objects consisting of attribute-value pairs. It’s commonly used to transmit data between a server and a web application or between different parts of an application.
A simple JSON object might look like this:
{
"employees": [
{
"name": "John Doe",
"position": "Manager"
},
{
"name": "Jane Smith",
"position": "Developer"
}
]
}
Reading JSON with PowerShell
PowerShell simplifies the process of reading JSON documents with the ConvertFrom-Json
cmdlet:
# Get JSON content
$jsonContent = Get-Content -Path "C:\path\to\your\file.json" -Raw
# Convert JSON to a PowerShell object
$jsonObject = $jsonContent | ConvertFrom-Json
# Access properties
$jsonObject.employees | ForEach-Object {
Write-Output ("Name: " + $_.name)
Write-Output ("Position: " + $_.position)
}
Creating JSON documents
Creating JSON with PowerShell is straightforward using the ConvertTo-Json
cmdlet:
# Create a hashtable
$hashtable = @{
employees = @(
@{
name = "John Doe"
position = "Manager"
},
@{
name = "Jane Smith"
position = "Developer"
}
)
}
# Convert the hashtable to JSON
$json = $hashtable | ConvertTo-Json -Depth 2
# Save JSON to a file
$json | Set-Content -Path "C:\path\to\your\file.json"
Modifying JSON documents
Modifying a JSON document involves reading the JSON, making changes to the resulting object, and then saving it back to JSON:
# Get JSON content and convert it to a PowerShell object
$jsonObject = Get-Content -Path "C:\path\to\your\file.json" -Raw | ConvertFrom-Json
# Modify the object
$jsonObject.employees[0].name = "New Name"
# Convert the object back to JSON and save it
$jsonObject | ConvertTo-Json -Depth 2 | Set-Content -Path "C:\path\to\your\file.json"
Best practices
Here are some best practices when working with JSON and PowerShell:
- Specify Depth When Converting to JSON: PowerShell’s
ConvertTo-Json
cmdlet only includes the first two levels of an object by default. Use the-Depth
parameter to include more levels if needed. - Be Mindful of Types: JSON does not distinguish between integers and floating-point numbers like PowerShell does. Be aware of this when converting between the two formats.
- Error Handling: Always include error handling when working with files or when parsing JSON.
The ability to work with JSON expands the versatility of your PowerShell scripts, allowing you to interact with modern APIs, configuration files, and more.
Leave a Reply