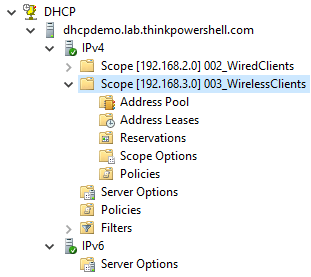
Use PowerShell to create dynamically assigned ranges of IP addresses using DHCP scopes.
What is a DHCP scope?
Per TechNet, a DHCP scope “is the consecutive range of possible IP addresses that the DHCP server can lease to clients on a subnet. Scopes typically define a single physical subnet on your network to which DHCP services are offered. Scopes are the primary way for the DHCP server to manage distribution and assignment of IP addresses and any related configuration parameters to DHCP clients on the network.”
Let’s say you have three subnets on your local network, 192.168.1.0/24, 192.168.2.0/24, and 192.168.3.0/24. The first subnet, 192.168.1.0/24, is a server and network management subnet where all IPs are statically assigned. For this subnet, you would not need to configure a DHCP scope.
For the other subnets, 192.168.2.0/24 is for your wired clients and 192.168.3.0/24 is for your wireless clients. These clients will connect and disconnect to the network throughout the day and week. For these subnets, you would configure a DHCP scope to handle the automatic assignment of IP address leases to these clients.
Creating a DHCP scope using PowerShell
In our previous post we installed the DHCP Server role and authorized it in our Active Directory domain. Building off of that configuration, we will create the DHCP scopes for 192.168.2.0/24 and 192.168.3.0/24.
For 192.168.2.0/24, here are the requirements for the DHCP scope:
- Assignable addresses should be between 192.168.2.21 and 192.168.2.250.
- The lease duration should be the default of 8 days.
- DHCP clients should be assigned 192.168.2.1 as their default gateway.
- DHCP clients should be assigned 192.168.1.11 as their DNS server.
- A TFTP server, 192.168.1.10, needs to be provided in the event a VoIP phone is connected.
Create the scope
We begin by creating the base IPv4 scope. Note the example below uses a technique called splatting to make the parameter set more readable. We will use the Add-DhcpServerv4Scope cmdlet:
# Create an IPv4 DHCP Server Scope $HashArgs = @{ 'Name' = '002_WiredClients'; 'Description' = 'Wired Clients'; 'StartRange' = '192.168.2.21'; 'EndRange' = '192.168.2.250'; 'SubnetMask' = '255.255.255.0'; 'State' = 'Active'; } Add-DhcpServerv4Scope @HashArgs
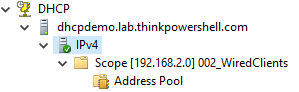
Set DHCP scope options
Now that we have created the scope, we can set the DHCP scope options. This includes things like (but are not limited to) the default gateway (aka ‘Router’), DNS servers, a domain name, and more.
Create a new server option
VoIP phones (e.g. a Cisco phone) requires this option be configured in order for the phone to get its configuration. By default, DHCP option 150 (TFTP server) is not available on a Windows DHCP Server. This option definition needs to be created.
# Create option definition for TFTP Server Add-DhcpServerv4OptionDefinition -OptionId 150 -Type IPv4Address -Name "TFTP Server"
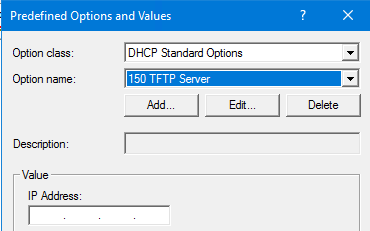
Set DHCP scope options
Now that we have created the scope and defined the additional option for a TFTP server, we can set the options for our scope using Set-DhcpServerv4OptionValue. Note that we use the ScopeId parameter to only set these options for this particular scope. If we were to omit this parameter, the option would be set at the server level, and would inherited by all scopes if they didn’t have the option explicitly set at the scope level.
# Set DHCP scope options $HashArgs = @{ 'ScopeId' = '192.168.2.0'; 'DnsServer' = '192.168.1.11'; 'DnsDomain' = 'lab.thinkpowershell.com'; 'Router' = '192.168.2.1'; } Set-DhcpServerv4OptionValue @HashArgs # Set TFTP option Set-DhcpServerv4OptionValue -ScopeId 192.168.2.0 -OptionId 150 -Value 192.168.1.10
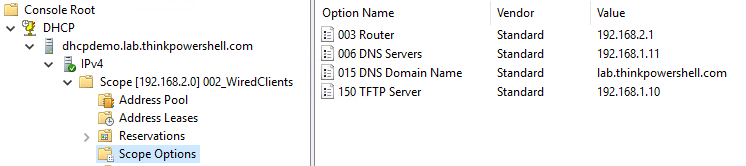
Create a DHCP Scope with a short lease duration
We have created the DHCP scope for wired clients, and now we want to create the scope for wireless clients. In our scenario, we know that we may see many different wireless clients in a given week, but most clients are only around for a single day at a time. I could expand the address range I allow for clients, but instead I am going to shorten the default lease duration to 1 day, so that addresses are only committed to a client for 24 hours at a time. I can use the LeaseDuration parameter and the format day.hrs:mins:secs to set that requirement.
# Create an IPv4 DHCP Server Scope $HashArgs = @{ 'Name' = '003_WirelessClients'; 'Description' = 'Wireless Clients'; 'StartRange' = '192.168.3.21'; 'EndRange' = '192.168.3.250'; 'SubnetMask' = '255.255.255.0'; 'LeaseDuration' = '1.00:00:00' # day.hrs:mins:secs 'State' = 'Active'; } Add-DhcpServerv4Scope @HashArgs # Set DHCP scope options $HashArgs = @{ 'ScopeId' = '192.168.3.0'; 'DnsServer' = '192.168.1.11'; 'DnsDomain' = 'lab.thinkpowershell.com'; 'Router' = '192.168.3.1'; } Set-DhcpServerv4OptionValue @HashArgs
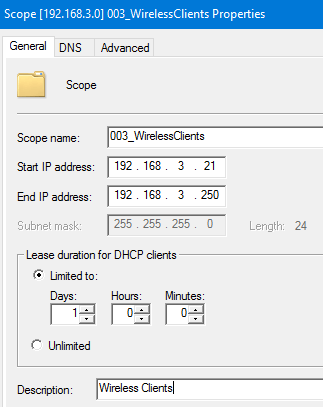
Copy DHCP scopes from an existing Windows DHCP Server
If you are already using a Windows DHCP server in your environment but are migrating to a new DHCP server, you can use PowerShell to copy the configured scopes from your old server to your new server:
# Copy DHCP scopes from one DHCP server to another Get-DhcpServerv4Scope -ComputerName OldDhcpServer | Add-DhcpServerv4Scope -ComputerName NewDhcpServer
Next Steps: Creating DHCP Reservations
DHCP is great for being able to avoid statically assigning IP addresses to devices, but how do you take advantage of the dynamic capability of DHCP, while still providing certain types of devices with a consistent, predictable address? In my next post, we will cover creating and migrating DHCP reservations.
Leave a Reply